[Full Stack] Single Page App (SPA) - ReactJs + Redux + Bootstrap 4 + ASP.Net Core

Coding On “Performance” Trend
From experience, a key requirement from stakeholders is always “performance”, and quite rightly so. Over the past 5 years there has been a large industry shift towards making use of Javascript libraries for creating powerful and beautiful user interfaces, but what if you need to maintain state and go full stack with ASP.Net Core on the server-side?
Today, we’re going to look at getting setup with a full stack single page application (SPA) implementation of ReactJS + Redux + Bootstrap 4 + ASP.Net Core.
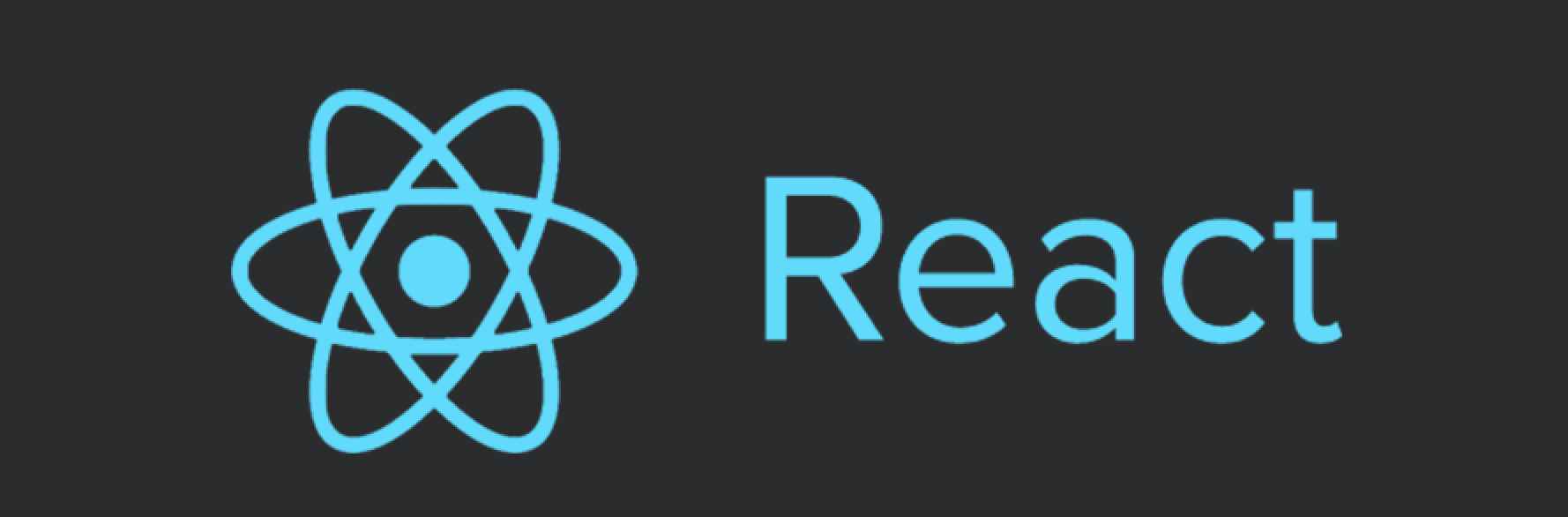
Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Enter “ReactJS”…
ReactJS was created by Jordan Walke, a software engineer at Facebook.
“ReactJS is a JavaScript library for building user interfaces. It is maintained by Facebook and a community of individual developers and companies.”
ReactJS makes heavy use of the Javascript syntax extension JSX (Javascript XML), which essentially makes Javascript look like Html written in a string, and is generally what components in React are written in, although they can be written in pure Javascript also.
ReactJS is component based, so only each component on a page needs to be updated. Remember AJAX? …imagine that with a more structured design and you’re halfway there.
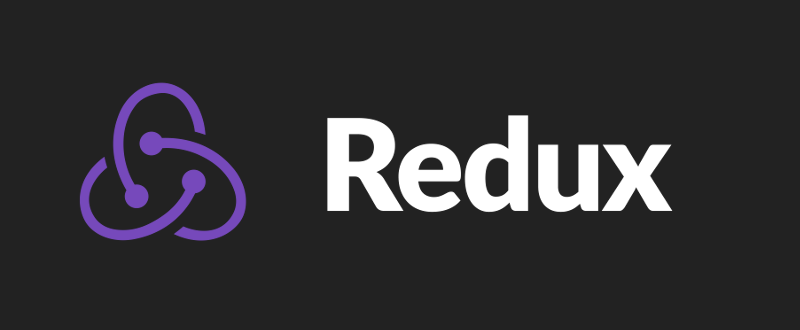
Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Enter “Redux”…
Redux is a “predictable state container” - It shares state across all components within an application. Redux has a store, which holds state for the entire application and the data is supplied as properties (this.props).
“Redux 4 has a much smaller footprint because they removed the lodash dependency (package management).”
Redux has three core components - Actions, Reducers, Store.
Actions - These are the payload of information that is sent from the application to the store. They are merely plain Javascript objects with the type defined as a string, and are created by an “action creator”.
Reducers - These specify how the state changes in response to an action. Reducers take the Action payload, and apply it to the store. The state is immutable, so a reducer returns a new state with whatever needs to be added, updated or removed.
Store - This holds the state and allows access to the state. The store allows the state to be updated, whilst also registering listeners to keep an eye on the state when it changes in different modules…
…essentially, Redux is quite powerful and extremely useful!
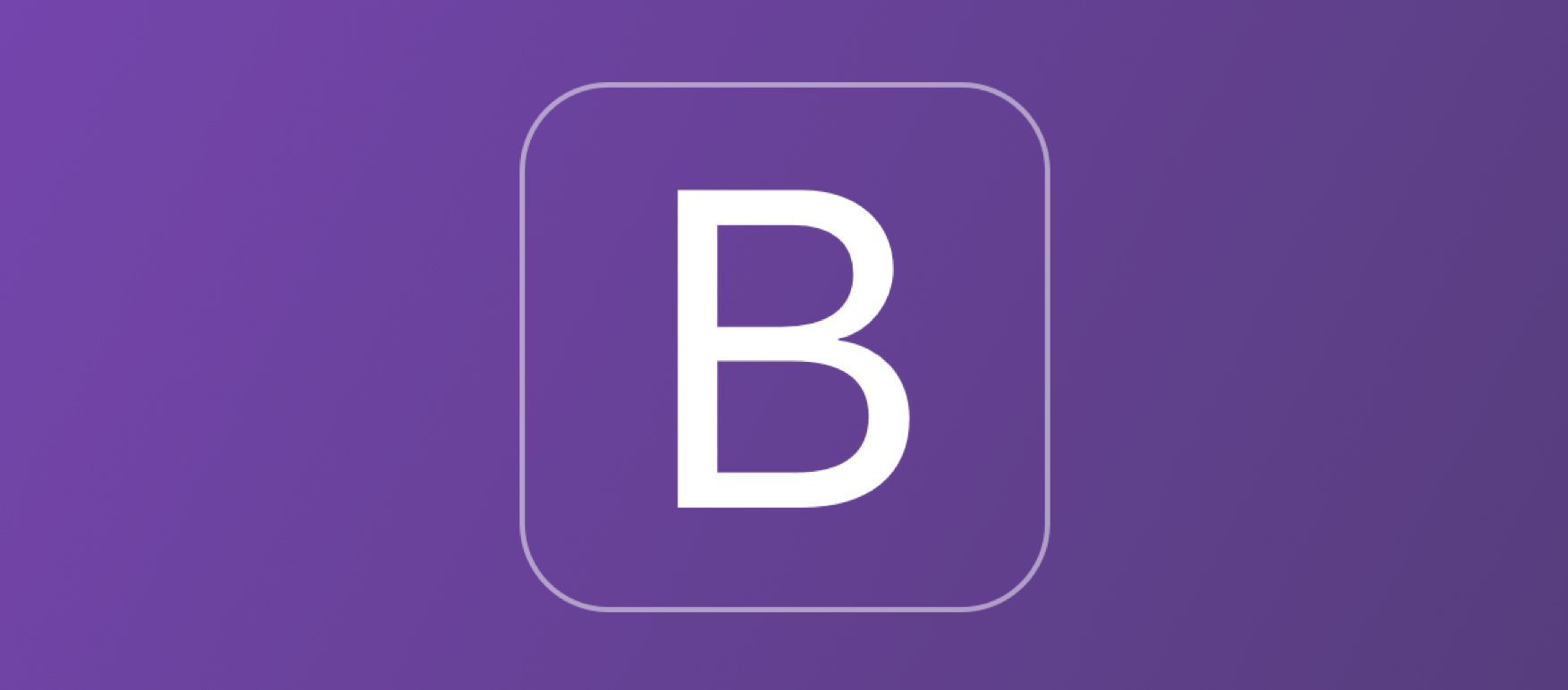
Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Enter “Bootstrap 4”…
I’m sure you know the Bootstrap story already - A mobile-responsive framework created by a dedicated team at Twitter originally in 2011 in order to maintain consistent implementations during front-end development. Bootstrap 3 was written in LESS, however Bootstrap 4 is written in SASS with the grid system based on REMs. It’s also worth noting that BS4 has a 64% smaller footprint than BS3 too. Bootstrap 4 is what we’ll be using today!
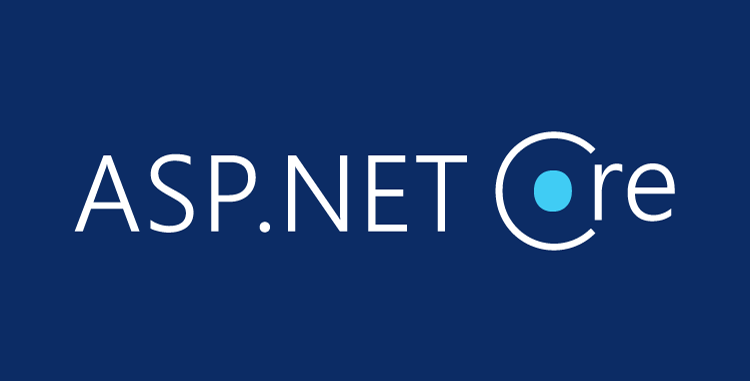
Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Enter “ASP.Net Core”…
Finally, our server-side framework will be running on ASP.Net Core. I’ll refrain from explaining this here, other than stating that it’s cross-platform and totally awesome!
Let’s Code…
First of all, you need to be running the latest version of Node on your machine.
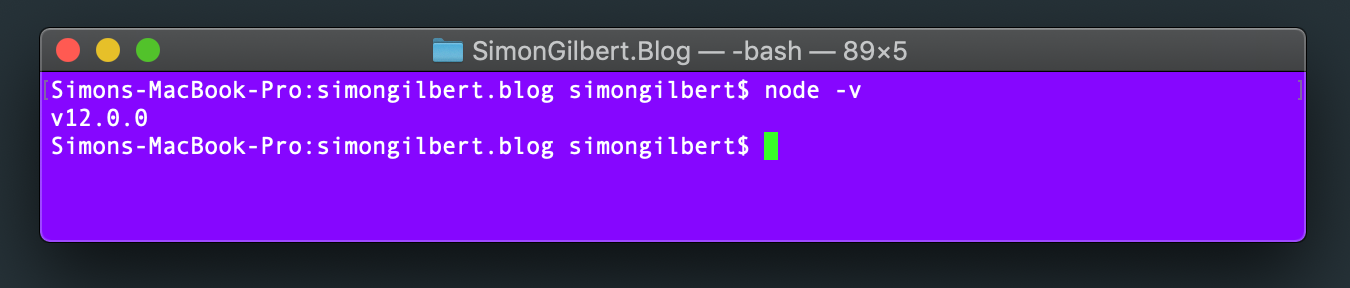
Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Next we’re going to use the relevant reactredux template to create our project, which for this example I’ve called “full-stack-app” (and this name must be entirely lowercase, but we can change it later).

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
We then need to CD into that directory to see the template files that have been created by our previous terminal statement.

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Once in that directory, open the .csproj file in Visual Studio (this generates a solution file). Save all, and close Visual Studio. You can then rename the solution and your .csproj file.
Following this, open the solution in Visual Studio, clean, rebuild. Then find and replace all of the original name (full-stack-app), to the new name (in my case SimonGilbert.Blog). When doing this, be sure to include both full-stack-app and the same name but with underscores i.e. full_stack_app.
Ok, now to upgrade some key packages. First, CD into the ClientApp subdirectory (this folder houses all the UI source code and packages).

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Let’s update the JQuery version.

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Now the prop-types package also.

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Followed by an upgrade to the latest version of React.

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
…including the React DOM.

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
And then Reactstrap (we’ll need this for Bootstrap usage later).

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Next we need to upgrade Redux to the latest version.

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Followed by Redux Thunk.

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
And finally, NPM audit fix…..to fix any issues that have occurred along the way (although some of the dependency errors can indeed be ignored).

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Reviewing Our Changes
If you now open the solution and navigate to the packages.json file, you should see something similar to this -
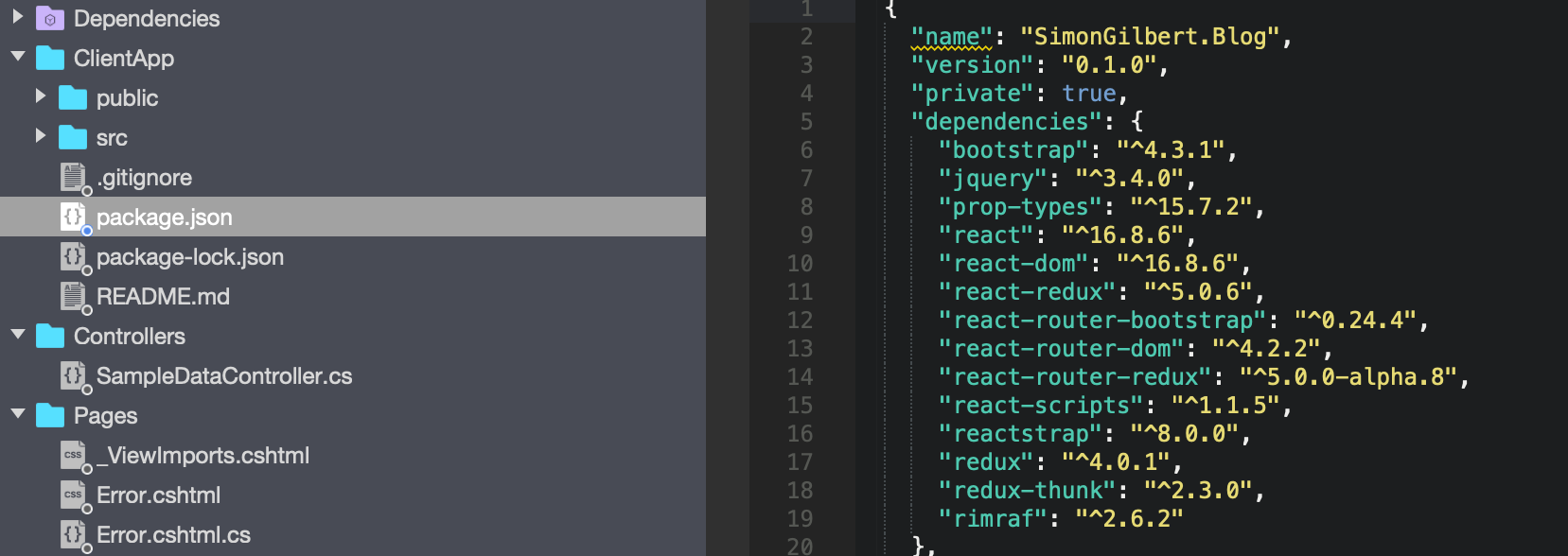
Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Running Our Application
Run the application as normal from Visual Studio, and you should see something similar to the following -
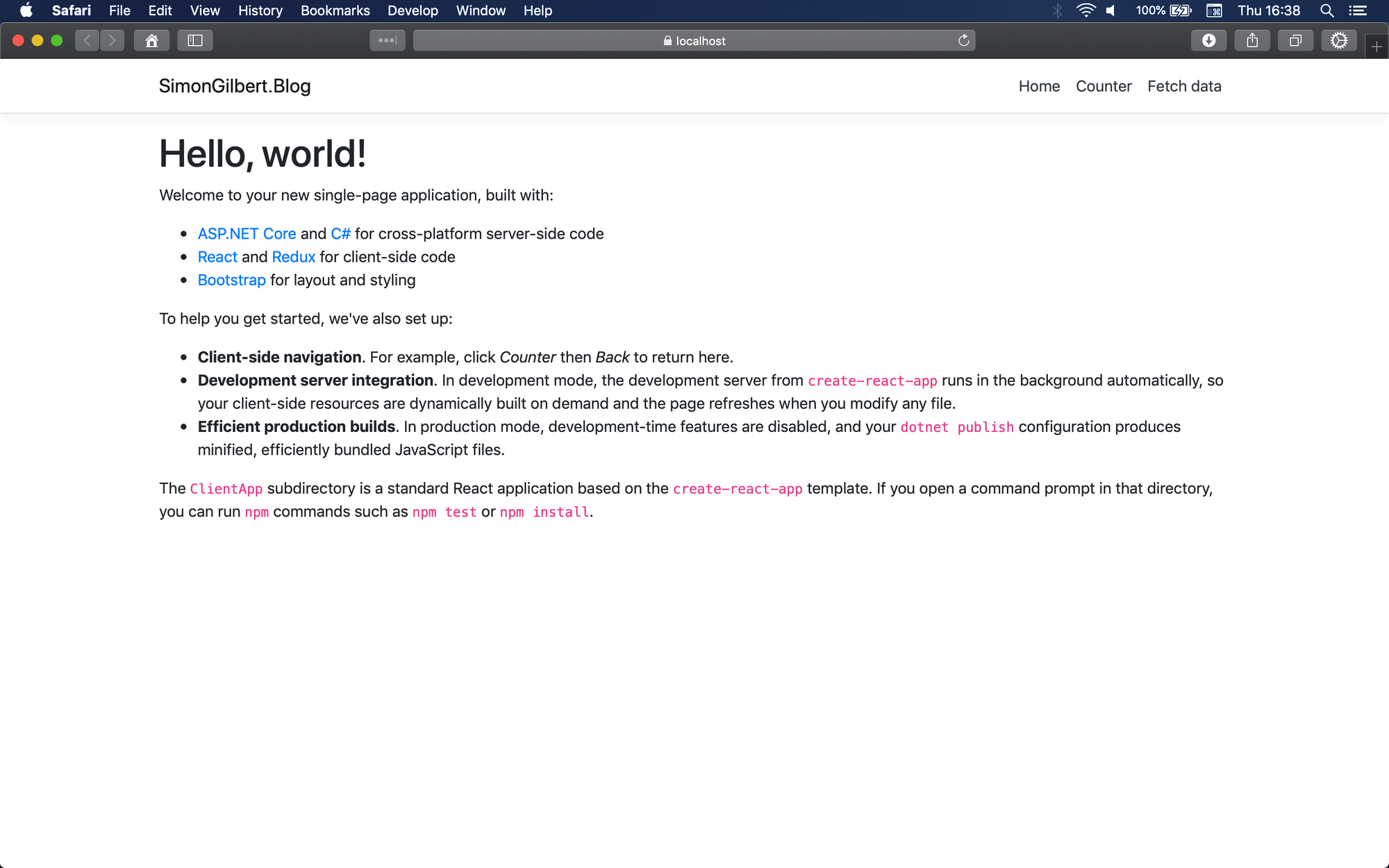
Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
…Result! Now head to the “Fetch data” tab which will load a series of weather forecast data from the server-side. If you now navigate o the controllers folder in Visual Studio, you’ll see the ASP.Net Core Web Api code endpoint that Redux is interacting with via ReactJS.

Full Stack Single Page App (SPA) Setup - ReactJs + Redux + Bootstrap 4 + ASP.Net Core
Enjoy!